Remote Configs
Introduction
Remote Configs package is designed to help your game studio gain insights into your player base and identify actionable opportunities for growing your audience and revenue.
The features that Remote Configs provides are,
Remote Configs automatically:
- Initialises selected Remote Configs solution (Satori or Firebase)
- Sets essential properties
- Logs important data (experiment name, etc.) to Analytics
- Fetches and stores Feature Flag values
Remote Configs also provides simple functions to:
- Access Feature Flag (remote configuration) values
- Fire Events (to assign payers to audiences)
- Set Custom Properties
Requirements
Remote Setup
Remote Configs solution has three types. Either of them can be configured in editor, but only one of them will be used at runtime. The three types of Remote Configs solution are:
Setup instructions for each solution are provided in the links above. After setting it up, you can select it by going to LionStudios → Settings Manager → Remote Configs
.
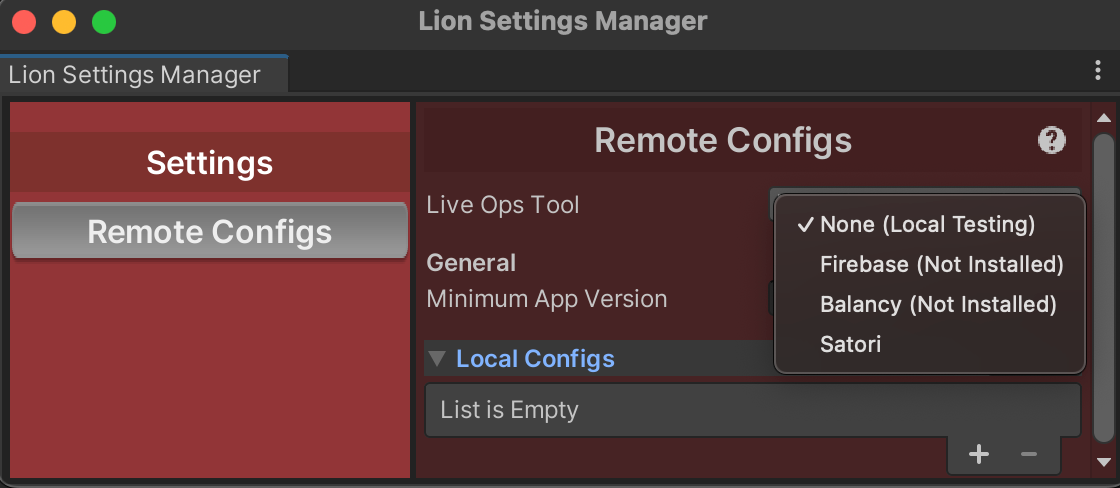
Loading Scene
The Remote Configs solutions need to load their values before the gameplay starts. The easiest solution for this is to integrate our Loading Scene module.
Usage
⚠️ | The following methods must be called after the Remote Configs package has been initialized.
The module is part of LionSDK’s initialization cycle; you can use If you have a loading scene already waiting for LionCore initialization, you don’t have to worry about this in your game scene. |
1. Remote values:
Remote values gives the ability to change the behaviour and appearance of your app without publishing an app update for users.
- For Firebase, Remote value means Remote configs
- For Satori, Remote value means Feature Flag
- For Balancy, Remote value means Template table.
- For Test, Remote value means local list of values present in editor window
Function signature
LiveopsController.GetValue<T>(
string flagName,
T defaultValue)
This will return the value of the feature flag for the current player.
Examples
// If no flag exists for that key or if offline, it will return the
// default value (3).
int showAppReviewAfterLevel =
LiveopsController.GetValue<int>("show_app_review_after_level", 3);
// Any type can be used. The flag value will be parsed to that type.
// For complex types, the flag value has to be formatted in json.
bool bonusLevelEnabled =
LiveopsController.GetValue<bool>("bonus_level_enabled", false);
⚠️ | Flag names are case-sensitive. |
2. Log Event
Events allow you to send data for a given user to the server for processing.
- For Firebase, the events are created on runtime so just need to call the “LogEvent” function with events.
- For satori, the event must be created in the Satori Dashboard for this function to work. See HeroicLabs Guides
- For Balancy, this function do nothing.
- For Test, the events are just Debug logs.
Function signature
LiveopsController.LogEvent(
string name,
string value,
Dictionary<string, string> metadata = null)
Example
// This will log an event named "iap_purchase", with a value of "verified"
LiveopsController.LogEvent("iap_purchase", "verified");
3. Fetching data
- For Firebase, By calling “FetchAndUpdateRemoteConfig” function, only remote configs are updated by fetching them from server.
- For Satori, By calling “FetchAndUpdateRemoteConfig” function, Remote configs and experiments are updated automatically as they are fetched again from the server.
- For Balancy, this function does nothing, as Balancy SDK already handles fetching data on it’s own.
- For Test, By calling “FetchAndUpdateRemoteConfig” function, will fetch latest values present in editor window. But in runtime this won’t do anything as values in unity editor will be the same everytime.
Function signature
The given function is an async task function, “await” can be used here, that ensures that code waits until the data is fetched completely from server.
LiveOpsController.FetchAndUpdateRemoteConfig();
Example
bool isFetchedCorrectly = await LiveOpsController.FetchAndUpdateRemoteConfig();
if (isFetchedCorrectly)
{
//Do something here after fetching
}
4. Custom/User properties
Properties are an important tool used to define and enrich a user identity profile, and ultimately used to define the audience segments.
- For Firebase, more information can be found here Firebase user properties
- For Satori, more information can be found here Satori Custom Properties
- For Balancy, more information can be found here Balancy Custom Properties and also here.
- For test, there are no user properties.Setting them won’t do anything.
Function signature
//Key parameter is the property id
//Value parameter is the property's actual value
public static void SetCustomProperty(string key, string value)
//Another way set properties is to send whole dictionary
//Dictionary's first 'string' denotes Key of property
//Dictionary's second 'string' denotes Value of property
public static void SetCustomProperties(Dictionary<string, string> customProperties)
Example-1
LiveOpsController.SetCustomProperty("Age", "23");
Example-2
Dictionary<string, string> dictionary = new Dictionary<string, string>()
{
{ "Age", "23"},
{ "Name", "Anything"}
};
LiveOpsController.SetCustomProperties(dictionary);
5. Existing user
A boolean value that’s used to identify players who have previously played the app in a version without Remote Configs (Satori or Firebase). These players must be processed differently internally because the Remote Configs SDK (Satori or Firebase) has incomplete data on them.
So at the start of the first session with Remote Configs, you need to let us know if the player has existed before that session. You can use your own persistent data to check this.
Make sure to call this only once.
Pass “true” if user existed before.
//Send boolean value as parameter that this is a new uwer or not.
public static void SetExistingUserProperty(bool value)
Example
if (!PlayerPrefs.HasKey("HasSetExistingUserProperty"))
{
bool userExistedBefore = PlayerPrefs.GetInt("CurrentLevel", 0) > 0;
LiveOpsController.SetExistingUserProperty(userExistedBefore);
PlayerPrefs.SetInt("HasSetExistingUserProperty", 1);
}
6. Set up experiments
Below are the instructions to set up AB Experiments on the dashboard so that the module can send appropriate events to Lion Analytics:
- For Firebase: AB Experiments (Firebase)
- For Satori: AB Experiments (Satori)
- For Balancy